You signed in with another tab or window. Reload to refresh your session.You signed out in another tab or window. Reload to refresh your session.You switched accounts on another tab or window. Reload to refresh your session.Dismiss alert
A controller or a service can have many underlying services to handle different requests. For example, ControllerA can have MyService and MyService can have ServiceA, ServiceB and so on.
The problem with this is all services are not used for some requests. an RequestForA request can only use ServiceA, or RequestForB can only use ServiceB. However, all services get initialized.
public class MyService : IMyService
{
private readonly IServiceA _serviceA;
private readonly IServiceB _serviceB;
public MyService(IServiceA serviceA, IServiceB serviceB)
{
_serviceA = serviceA;
_serviceB = serviceB;
}
public void DoWorkA()
{
_serviceA.DoWork();
}
public void DoWorkB()
{
_serviceB.DoWork();
}
}
Describe the solution you'd like
One solution is to Singleton, so all initialization overhead get mitigated. However, doing so forces us to pass down request-only properties into method where we could store in request-only object and inject into services.
Or we can have a lazy initialization like this
[RegisterPerRequest(supportLazyInitialization: true)]
public class ServiceA : IServiceA
{
public void DoWork(int value)
{
// do something for A
}
}
public class MyService : IMyService
{
private readonly Lazy<IServiceA> _serviceA;
private readonly Lazy<IServiceB> _serviceB;
public MyService(Lazy<IServiceA> serviceA, Lazy<IServiceB> serviceB)
{
_serviceA = serviceA;
_serviceB = serviceB;
}
public void DoWork(int value)
{
if (value < 42)
{
_serviceA.Value.DoWork();
}
else
{
_serviceB.Value.DoWork();
}
}
}
// Registering a type mapping for the lazy proxy.
builder
.Register(c =>
{
var context = c.Resolve<IComponentContext>();
return LazyProxyBuilder.CreateInstance(
() => context.ResolveNamed<IMyService>(registrationName));
})
.As<IMyService>();
As long as we're taking about controllers - controller actions can mark their parameters as [FromServices] (details link). This does not solve dependencies of "general" (non-controller) classes, nor the very niche case mentioned in your referred article, where we have different dependencies based on branches.
I wonder, do you have an example to show where this feature would actually provide benefits - and this is actually the right way to achieve that (as opposed to refactoring)? I'm NOT against the idea, just trying to understand use-cases.
Feature request
Type
Is your feature request related to a problem?
A controller or a service can have many underlying services to handle different requests. For example, ControllerA can have MyService and MyService can have ServiceA, ServiceB and so on.
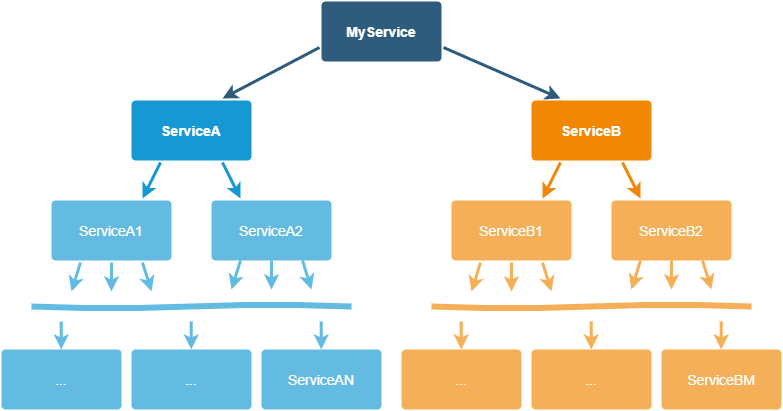
The problem with this is all services are not used for some requests. an RequestForA request can only use ServiceA, or RequestForB can only use ServiceB. However, all services get initialized.
Describe the solution you'd like
One solution is to Singleton, so all initialization overhead get mitigated. However, doing so forces us to pass down request-only properties into method where we could store in request-only object and inject into services.
Or we can have a lazy initialization like this
Additional context
The text was updated successfully, but these errors were encountered: