Chart.js plugin for adding error bars to Line-, Barcharts or hierarchical Barcharts. This plugin also works with the Hierarchical Scale Plugin.
Try the demo on Codepen.
npm install --save chart.js chartjs-plugin-error-bars
Datasets must define an errorBars
object that contains the error bar property key (same as in the used scale) and values plus
and minus
. Plus values are always positive, and minus vice versa.
Categorical scale usage:
data: {
labels: ['January', 'February', 'March', 'April', 'May', 'June', 'July'],
datasets: [{
...
errorBars: {
'February': {plus: 15, minus: -34},
'March': {plus: 5, minus: -24},
'May': {plus: 35, minus: -14},
'June': {plus: 45, minus: -4}
}, ...
/* or for graded error bars
errorBars: {
'February': [{plus: 15, minus: -34}, {plus: 10, minus: -26}],
'March': [{plus: 5, minus: -24}, {plus: 2, minus: -16}],
'May': [{plus: 35, minus: -14}, {plus: 7, minus: -7}],
'June': [{plus: 45, minus: -4}, {plus: 25, minus: -2}]
}, ...
*/
corresponding TypeScript interface
interface IErrorBars {
[label: string]: IErrorBar | IErrorBar[];
}
interface IErrorBar {
plus: number;
minus: number;
}
Hierarchical scale plugin usage:
data: {
labels: [
'A',
{
label: 'C1',
children: ['C1.1', 'C1.2', 'C1.3', 'C1.4']
}
],
datasets: [{
...
errorBars: {
'A': {plus: 25, minus: -10},
'C1.2': {plus: 14, minus: -15},
'C1': {plus: 34, minus: -5}
}, ...
}
Find more Samples on Github.
options: {
...
plugins: {
chartJsPluginErrorBars: {
/**
* stroke color, or array of colors
* @default: derived from borderColor
*/
color: '#666',
/**
* bar width in pixel as number or string or bar width in percent based on the barchart bars width (max 100%), or array of such definition
* @default 10
*/
width: 10 | '10px' | '60%',
/**
* lineWidth as number, or as string with pixel (px) ending
*/
lineWidth: 2 | '2px',
/**
* lineWidth as number, or as string with pixel (px) ending, or array of such definition
* @default 2
*/
lineWidth: 2 | '2px',
/**
* whether to interpet the plus/minus values, relative to the value itself (default) or absolute
* @default false
*/
absoluteValues: false
}
}
...
}
corresponding TypeScript interface
interface IChartJsPluginErrorBarsOptions {
color: string | string[];
width: (string | number) | (string | number)[];
lineWidth: (string | number) | (string | number)[];
absoluteValues: boolean;
}
npm install
npm run build
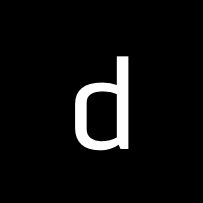