-
Notifications
You must be signed in to change notification settings - Fork 0
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
Showing
10 changed files
with
184 additions
and
0 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,10 @@ | ||
#1. Create a greeting for your program. | ||
print("Welcome to the Generator") | ||
#2. Ask the user for the city that they grew up in. | ||
city = input("What is name of the city you grew up in\n ") | ||
#3. Ask the user for the name of a pet. | ||
pet = input("What is name of pet? \n") | ||
#4. Combine the name of their city and pet and show them their band name. | ||
print("Band Name: " + city + " " + pet) | ||
#5. Make sure the input cursor shows on a new line, see the example at: | ||
# https://band-name-generator-end.appbrewery.repl.run/ |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,34 @@ | ||
## Debugging | ||
|
||
# Instructions | ||
|
||
Look at the code in the code editor on the left. There are errors in all of the lines of code. Fix the code so that it runs without errors. | ||
|
||
**Warning:** The output in your program should match the example output shown below exactly, character for character, even spaces and symbols should be identical, otherwise the tests won't pass. | ||
|
||
# Example Output | ||
|
||
When you run your program, it should print the following: | ||
|
||
``` | ||
Day 1 - String Manipulation | ||
String Concatenation is done with the "+" sign. | ||
e.g. print("Hello " + "world") | ||
New lines can be created with a backslash and n. | ||
``` | ||
|
||
e.g. When you hit **run**, there should be no errors and this is what should happen: | ||
|
||
 | ||
|
||
# Test Your Code | ||
|
||
Before checking the solution, try copy-pasting your code into this repl: | ||
|
||
[https://repl.it/@appbrewery/day-1-2-test-your-code](https://repl.it/@appbrewery/day-1-2-test-your-code) | ||
|
||
This repl includes my testing code that will check if your code meets this assignment's objectives. | ||
|
||
# Solution | ||
|
||
[https://repl.it/@appbrewery/day-1-2-solution](https://repl.it/@appbrewery/day-1-2-solution) |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,8 @@ | ||
#Fix the code below π | ||
|
||
print("Day 1 - String Manipulation") | ||
print("String Concatenation is done with the "+" sign.") | ||
print('e.g. print("Hello " + "world")') | ||
print("New lines can be created with a backslash and n.") | ||
|
||
|
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,45 @@ | ||
## Inputs | ||
|
||
# Instructions | ||
|
||
Write a program that prints the number of characters in a user's name. You might need to Google for a function that calculates the length of a string. | ||
|
||
e.g. | ||
|
||
[https://www.google.com/search?q=how+to+get+the+length+of+a+string+in+python+stack+overflow](https://www.google.com/search?sxsrf=ACYBGNRxEaJIWyKHuWI0Lk24t4KuZVyeew:1579706585702&q=how+to+get+the+length+of+a+string+in+python+stack+overflow) | ||
|
||
**Warning.** Your program should work for different inputs. e.g. any name that you input. | ||
|
||
# Example Input | ||
|
||
``` | ||
Angela | ||
``` | ||
|
||
# Example Output | ||
|
||
``` | ||
6 | ||
``` | ||
|
||
e.g. When you hit **run**, this is what should happen: | ||
|
||
 | ||
|
||
# Hint | ||
|
||
1. You can put functions inside other functions. | ||
2. Don't try to print anything other than the length. | ||
|
||
# Test Your Code | ||
|
||
Before checking the solution, try copy-pasting your code into this repl: | ||
|
||
[https://repl.it/@appbrewery/day-1-3-test-your-code](https://repl.it/@appbrewery/day-1-3-test-your-code) | ||
|
||
This repl includes my testing code that will check if your code meets this assignment's objectives. | ||
|
||
|
||
# Solution | ||
|
||
[https://repl.it/@appbrewery/day-1-3-solution](https://repl.it/@appbrewery/day-1-3-solution) |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,11 @@ | ||
#Write your code below this line π | ||
|
||
a = input("Input User name: ") | ||
print(len(a)) | ||
|
||
|
||
|
||
|
||
|
||
|
||
|
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,51 @@ | ||
## Variables | ||
|
||
# Instructions | ||
|
||
Write a program that switches the values stored in the variables a and b. | ||
|
||
**Warning.** Do not change the code on lines 1-4 and 12-18. Your program should work for different inputs. e.g. any value of a and b. | ||
|
||
# Example Input | ||
|
||
``` | ||
a: 3 | ||
``` | ||
|
||
``` | ||
b: 5 | ||
``` | ||
|
||
# Example Output | ||
|
||
``` | ||
a: 5 | ||
``` | ||
|
||
``` | ||
b: 3 | ||
``` | ||
|
||
e.g. When you hit **run**, this is what should happen: | ||
|
||
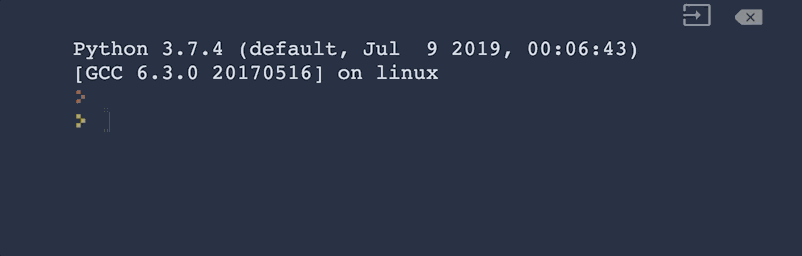 | ||
|
||
# Hint | ||
|
||
1. You should not have to type any numbers in your code. | ||
2. You might need to make some more variables. | ||
|
||
# Test Your Code | ||
|
||
Before checking the solution, try copy-pasting your code into this repl: | ||
|
||
[https://repl.it/@appbrewery/day-1-4-test-your-code](https://repl.it/@appbrewery/day-1-4-test-your-code) | ||
|
||
This repl includes my testing code that will check if your code meets this assignment's objectives. | ||
|
||
|
||
|
||
|
||
# Solution | ||
|
||
[https://repl.it/@appbrewery/day-1-4-solution](https://repl.it/@appbrewery/day-1-4-solution) |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,20 @@ | ||
# π¨ Don't change the code below π | ||
a = input("a: ") | ||
b = input("b: ") | ||
# π¨ Don't change the code above π | ||
|
||
#################################### | ||
#Write your code below this line π | ||
|
||
temp = a | ||
a = b | ||
b = temp | ||
|
||
#Write your code above this line π | ||
#################################### | ||
|
||
# π¨ Don't change the code below π | ||
print("a: " + a) | ||
print("b: " + b) | ||
|
||
|
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,3 @@ | ||
# input() will get user input in console | ||
# Then print() will print the word "Hello" and the user input | ||
print("Hello " + input("What is your name?")) |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,2 @@ | ||
# Write your code below this line π | ||
print("Hello World!") |
Empty file.