LightDSA is a lightweight digital signature algorithm library for Python. It is a hybrid library supporting Elliptic Curve Digital Signature Algorithm (ECDSA) and Edwards-Curve Digital Signature Algorithm (EdDSA), RSA and DSA.
The easiest way to install the LightDSA package is to install it from python package index (PyPI).
pip install lightdsa
Then you will be able to import the library and use its functionalities.
from lightdsa import LightDSA
Once you initialize a LightDSA object with a specific DSA algorithm, such as ECDSA, EdDSA, or RSA, it will generate the private and public keys for your cryptosystem.
# built a cryptosystem
dsa = LightDSA(algorithm_name = "eddsa") # or ecdsa, rsa
# export keys
dsa.export_keys("public.txt", public = True)
# sign a message
message = "Hello, world!"
signature = dsa.sign(message)
If you wish to use a pre-existing exported cryptosystem, you should specify the exported key file during initialization. This will allow you to sign messages using the private key and verify messages using the public key as
# restore the cryptosystem for the verifier side
verifier_dsa = LightDSA(algorithm_name = "eddsa", key_file = "public.txt")
verifier_dsa.verify(message, signature)
LightDSA supports Weierstrass, Koblitz and Edwards forms and hundreds of pre-defined curves that can be adopted in ECDSA and EdDSA.
By default, ECDSA uses the Weierstrass form with the secp256k1 curve (the curve used by Bitcoin), while EdDSA uses the Edwards form with the ed25519 curve. However, you can switch the elliptic curve form and the specific curve for both ECDSA and EdDSA during the initialization of the LightDSA object. Meanwhile, the hashing algorithm is determined based on the order of the elliptic curve, which defines the number of points available over the finite field.
dsa = LightDSA(
algorithm_name = "eddsa",
form_name = "edwards",
curve_name = "ed448"
)
See curves
page for more details.
All PRs are more than welcome! If you are planning to contribute a large patch, please create an issue first to get any upfront questions or design decisions out of the way first.
You should be able run make test
and make lint
commands successfully before committing. Once a PR is created, GitHub test workflow will be run automatically and unit test results will be available in GitHub actions before approval.
There are many ways to support a project - starring⭐️ the GitHub repo is just one 🙏
You can also support this work on Patreon, GitHub Sponsors or Buy Me a Coffee.
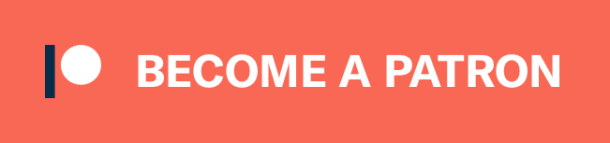
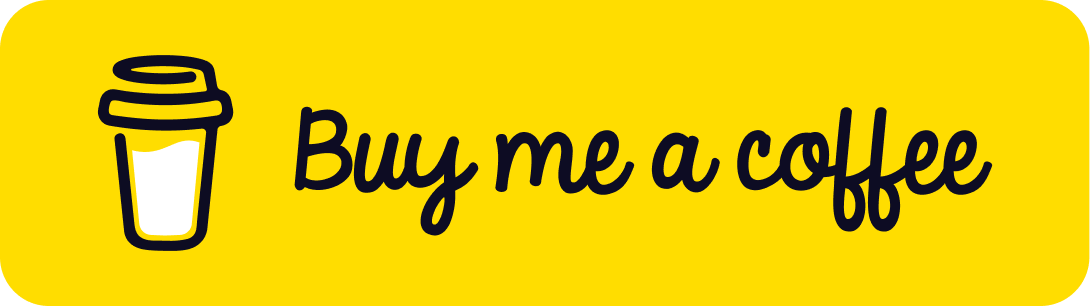
Also, your company's logo will be shown on README on GitHub if you become sponsor in gold, silver or bronze tiers.
Please cite LightDSA in your publications if it helps your research. Here is its BibTex entry:
@misc{serengil2025lightdsa
author = {Serengil, Sefik Ilkin},
title = {LightDSA: A Lightweight Digital Signature Algorithm Library for Python},
year = {2025},
publisher = {GitHub},
howpublished = {https://github.com/serengil/LightDSA},
}
LightDSA is licensed under the MIT License - see LICENSE
for more details.
LightDSA logo is created by M. Ristiyanto and it is licensed under Creative Commons: By Attribution 3.0 License.