-
Notifications
You must be signed in to change notification settings - Fork 24
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
Showing
13 changed files
with
1,087 additions
and
0 deletions.
There are no files selected for viewing
Loading
Sorry, something went wrong. Reload?
Sorry, we cannot display this file.
Sorry, this file is invalid so it cannot be displayed.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,92 @@ | ||
# QR Detection with OpenCV and YOLO Model in Python | ||
This repository provides samples demonstrating how to detect QR codes using **YOLO** and how to read QR codes with the [Dynamsoft Barcode Reader](https://www.dynamsoft.com/barcode-reader/overview/). | ||
|
||
## Prerequisites | ||
- OpenCV 4.x | ||
|
||
``` | ||
pip install opencv-python | ||
``` | ||
- Dynamsoft Barcode Reader | ||
``` | ||
pip install dbr | ||
``` | ||
- Obtain a [Dynamsoft Barcode Reader trial license](https://www.dynamsoft.com/customer/license/trialLicense?product=dbr) and update your code with the provided license key: | ||
```python | ||
from dbr import * | ||
license_key = "LICENSE-KEY" | ||
BarcodeReader.init_license(license_key) | ||
reader = BarcodeReader() | ||
``` | ||
## Usage | ||
#### QR Detection | ||
- From Image File: | ||
``` | ||
python3 opencv-yolo.py | ||
``` | ||
- From Camera: | ||
``` | ||
python3 opencv-yolo-camera.py | ||
``` | ||
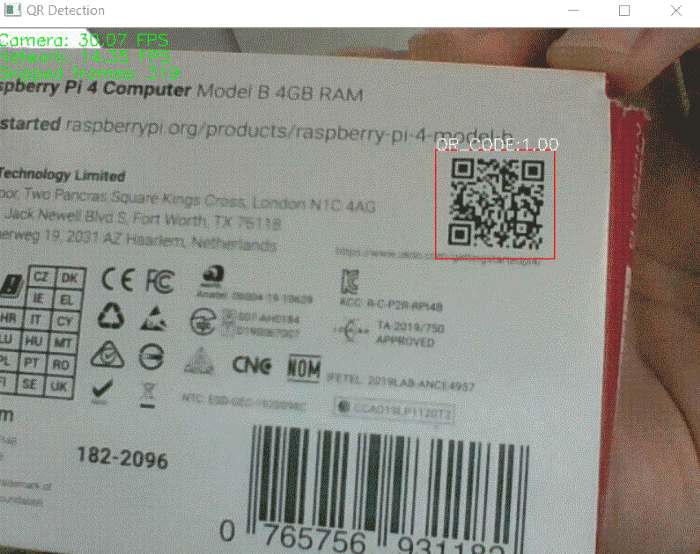 | ||
#### QR Reading with Dynamsoft Barcode Reader | ||
Below is a sample code snippet for reading QR codes with the Dynamsoft Barcode Reader: | ||
```py | ||
from dbr import * | ||
license_key = "LICENSE-KEY" | ||
BarcodeReader.init_license(license_key) | ||
reader = BarcodeReader() | ||
settings = reader.reset_runtime_settings() | ||
settings = reader.get_runtime_settings() | ||
settings.region_bottom = bottom | ||
settings.region_left = left | ||
settings.region_right = right | ||
settings.region_top = top | ||
reader.update_runtime_settings(settings) | ||
try: | ||
text_results = reader.decode_buffer(frame) | ||
if text_results != None: | ||
for text_result in text_results: | ||
print("Barcode Format :") | ||
print(text_result.barcode_format_string) | ||
print("Barcode Text :") | ||
print(text_result.barcode_text) | ||
print("Localization Points : ") | ||
print(text_result.localization_result.localization_points) | ||
print("-------------") | ||
except BarcodeReaderError as bre: | ||
print(bre) | ||
``` | ||
|
||
- From Image File: | ||
|
||
``` | ||
python3 yolo-dbr.py | ||
``` | ||
- From Camera: | ||
``` | ||
python3 yolo-dbr-camera.py | ||
``` | ||
## Blog | ||
[How to Detect and Decode QR Code with YOLO, OpenCV, and Dynamsoft Barcode Reader](https://www.dynamsoft.com/codepool/qr-code-detect-decode-yolo-opencv.html) |
Loading
Sorry, something went wrong. Reload?
Sorry, we cannot display this file.
Sorry, this file is invalid so it cannot be displayed.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,145 @@ | ||
from time import sleep | ||
import cv2 as cv | ||
import numpy as np | ||
import time | ||
from threading import Thread | ||
import queue | ||
from dbr import * | ||
|
||
license_key = "DLS2eyJoYW5kc2hha2VDb2RlIjoiMjAwMDAxLTE2NDk4Mjk3OTI2MzUiLCJvcmdhbml6YXRpb25JRCI6IjIwMDAwMSIsInNlc3Npb25QYXNzd29yZCI6IndTcGR6Vm05WDJrcEQ5YUoifQ==" | ||
BarcodeReader.init_license(license_key) | ||
reader = BarcodeReader() | ||
color = (0, 0, 255) | ||
thickness = 2 | ||
|
||
|
||
def decodeframe(frame): | ||
|
||
try: | ||
outs = reader.decode_buffer(frame) | ||
if outs != None: | ||
return outs | ||
except BarcodeReaderError as bre: | ||
print(bre) | ||
|
||
return None | ||
|
||
|
||
winName = 'QR Detection' | ||
|
||
|
||
def postprocess(frame, outs): | ||
if outs == None: | ||
return | ||
|
||
for out in outs: | ||
points = out.localization_result.localization_points | ||
|
||
cv.line(frame, points[0], points[1], color, thickness) | ||
cv.line(frame, points[1], points[2], color, thickness) | ||
cv.line(frame, points[2], points[3], color, thickness) | ||
cv.line(frame, points[3], points[0], color, thickness) | ||
cv.putText(frame, out.barcode_text, (min([point[0] for point in points]), min( | ||
[point[1] for point in points])), cv.FONT_HERSHEY_SIMPLEX, 1, color, thickness) | ||
|
||
|
||
cap = cv.VideoCapture(0) | ||
|
||
|
||
class QueueFPS(queue.Queue): | ||
def __init__(self): | ||
queue.Queue.__init__(self) | ||
self.startTime = 0 | ||
self.counter = 0 | ||
|
||
def put(self, v): | ||
queue.Queue.put(self, v) | ||
self.counter += 1 | ||
if self.counter == 1: | ||
self.startTime = time.time() | ||
|
||
def getFPS(self): | ||
return self.counter / (time.time() - self.startTime) | ||
|
||
|
||
process = True | ||
|
||
# | ||
# Frames capturing thread | ||
# | ||
framesQueue = QueueFPS() | ||
|
||
|
||
def framesThreadBody(): | ||
global framesQueue, process | ||
|
||
while process: | ||
hasFrame, frame = cap.read() | ||
if not hasFrame: | ||
break | ||
framesQueue.put(frame) | ||
|
||
|
||
# | ||
# Frames processing thread | ||
# | ||
decodingQueue = QueueFPS() | ||
|
||
|
||
def processingThreadBody(): | ||
global decodingQueue, process | ||
|
||
while process: | ||
# Get a next frame | ||
frame = None | ||
try: | ||
frame = framesQueue.get_nowait() | ||
framesQueue.queue.clear() | ||
except queue.Empty: | ||
pass | ||
|
||
if not frame is None: | ||
outs = decodeframe(frame) | ||
decodingQueue.put((frame, outs)) | ||
sleep(0.03) | ||
|
||
|
||
framesThread = Thread(target=framesThreadBody) | ||
framesThread.start() | ||
|
||
processingThread = Thread(target=processingThreadBody) | ||
processingThread.start() | ||
|
||
# | ||
# Postprocessing and rendering loop | ||
# | ||
while cv.waitKey(1) < 0: | ||
try: | ||
# Request prediction first because they put after frames | ||
outs = decodingQueue.get_nowait() | ||
frame = outs[0] | ||
postprocess(frame, outs[1]) | ||
|
||
# Put efficiency information. | ||
if decodingQueue.counter > 1: | ||
label = 'Camera: %.2f FPS' % (framesQueue.getFPS()) | ||
cv.putText(frame, label, (0, 15), | ||
cv.FONT_HERSHEY_SIMPLEX, 0.5, (0, 255, 0)) | ||
|
||
label = 'DBR SDK: %.2f FPS' % (decodingQueue.getFPS()) | ||
cv.putText(frame, label, (0, 30), | ||
cv.FONT_HERSHEY_SIMPLEX, 0.5, (0, 255, 0)) | ||
|
||
label = 'Skipped frames: %d' % ( | ||
framesQueue.counter - decodingQueue.counter) | ||
cv.putText(frame, label, (0, 45), | ||
cv.FONT_HERSHEY_SIMPLEX, 0.5, (0, 255, 0)) | ||
|
||
cv.imshow(winName, frame) | ||
except queue.Empty: | ||
pass | ||
|
||
|
||
process = False | ||
framesThread.join() | ||
processingThread.join() |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,45 @@ | ||
import cv2 as cv | ||
import numpy as np | ||
import time | ||
from dbr import * | ||
|
||
license_key = "DLS2eyJoYW5kc2hha2VDb2RlIjoiMjAwMDAxLTE2NDk4Mjk3OTI2MzUiLCJvcmdhbml6YXRpb25JRCI6IjIwMDAwMSIsInNlc3Npb25QYXNzd29yZCI6IndTcGR6Vm05WDJrcEQ5YUoifQ==" | ||
BarcodeReader.init_license(license_key) | ||
reader = BarcodeReader() | ||
color = (0, 0, 255) | ||
thickness = 2 | ||
|
||
|
||
def decodeframe(frame): | ||
|
||
try: | ||
text_results = reader.decode_buffer(frame) | ||
|
||
if text_results != None: | ||
for text_result in text_results: | ||
print("Barcode Format :") | ||
print(text_result.barcode_format_string) | ||
print("Barcode Text :") | ||
print(text_result.barcode_text) | ||
print("Localization Points : ") | ||
print(text_result.localization_result.localization_points) | ||
print("-------------") | ||
points = text_result.localization_result.localization_points | ||
|
||
cv.line(frame, points[0], points[1], color, thickness) | ||
cv.line(frame, points[1], points[2], color, thickness) | ||
cv.line(frame, points[2], points[3], color, thickness) | ||
cv.line(frame, points[3], points[0], color, thickness) | ||
|
||
cv.putText(frame, text_result.barcode_text, (min([point[0] for point in points]), min( | ||
[point[1] for point in points])), cv.FONT_HERSHEY_SIMPLEX, 1, color, thickness) | ||
except BarcodeReaderError as bre: | ||
print(bre) | ||
|
||
|
||
# Load an frame | ||
frame = cv.imread("416x416.jpg") | ||
decodeframe(frame) | ||
|
||
cv.imshow('QR Detection', frame) | ||
cv.waitKey() |
Oops, something went wrong.